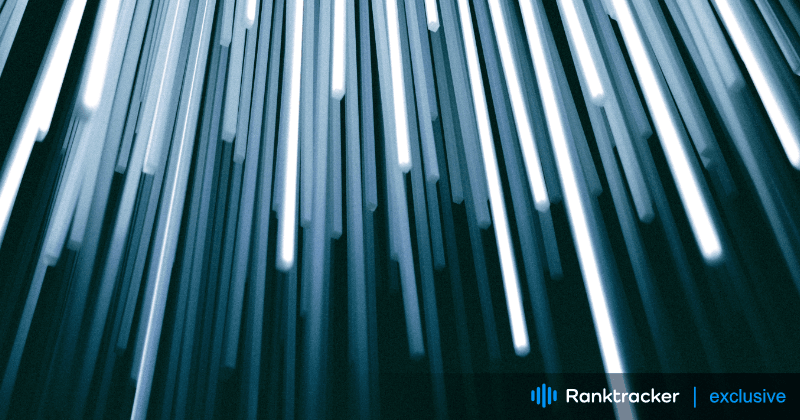
Intro
Next.js is a popular React framework known for its versatility in creating both static and dynamic websites with server-side rendering (SSR) and static site generation (SSG). With built-in features like automatic code splitting, image optimization, and fast page loading, Next.js is ideal for building SEO-friendly websites. However, to fully optimize your Next.js SEO, you need to implement specific strategies that enhance search engine visibility and performance.
In this guide, we’ll explore how to optimize SEO for your Next.js site, focusing on technical and on-page SEO techniques, performance optimizations, and best practices to ensure your site ranks well in search engine results pages (SERPs).
Why SEO is Important for Next.js Sites
Next.js provides a solid foundation for SEO through its support for server-side rendering (SSR) and static site generation (SSG), both of which improve how search engines crawl and index content. However, achieving high rankings requires more than just leveraging Next.js’s default capabilities. Effective SEO ensures that search engines understand your content, leading to higher visibility, increased traffic, and better user engagement.
Key benefits of optimizing Next.js SEO include:
-
Higher search rankings: Optimized content ranks better on Google and other search engines.
-
Improved user experience: Faster load times, optimized metadata, and responsive design enhance user engagement and reduce bounce rates.
-
Increased organic traffic: Proper SEO increases your site’s discoverability, leading to more visitors and conversions.
Key SEO Considerations for Next.js
1. Server-Side Rendering (SSR) and Static Site Generation (SSG)
One of the main reasons Next.js is SEO-friendly is its ability to support both SSR and SSG. These rendering methods make it easier for search engines to crawl and index content, improving your chances of ranking.
-
Server-Side Rendering (SSR): With SSR, Next.js generates the HTML on the server for each request. This ensures that search engines can crawl fully rendered HTML, rather than waiting for JavaScript to load content.
-
Static Site Generation (SSG): SSG pre-builds pages at build time into static HTML files. Static pages are lightweight and load extremely fast, which helps with SEO performance.
Use SSR for dynamic pages that require real-time data, such as product pages, and SSG for static content like blogs or marketing pages to maximize SEO benefits.
2. Title Tags, Meta Descriptions, and Headers
On-page SEO elements like title tags, meta descriptions, and header tags help search engines understand the structure and content of your pages. In Next.js, you can easily manage these elements using the Head component from next/head
.
-
Title Tags: Ensure each page has a unique and keyword-rich title tag, limited to around 60 characters. This helps search engines and users understand the main topic of the page.
-
Meta Descriptions: Add meta descriptions for each page, summarizing the content and including relevant keywords. Meta descriptions should be 150-160 characters to ensure full visibility in search results.
-
Header Tags (H1, H2, etc.): Use structured headers to organize your content logically. The H1 tag should contain your primary keyword, and subheadings (H2, H3) should provide further structure.
Example usage in Next.js:
import Head from 'next/head';
export default function Home() {
return (
<>
<Head>
<title>Next.js SEO Optimization | Improve Your Site Ranking</title>
<meta name="description" content="Learn how to optimize your Next.js site for SEO to improve search engine rankings." />
</Head>
<h1>Next.js SEO Optimization Guide</h1>
{/* Rest of your page content */}
</>
);
}
Ranktracker’s SEO Audit tool can help identify missing or incorrectly configured meta tags and headers across your Next.js site, ensuring that each page is fully optimized.
3. Image Optimization
Next.js has built-in support for image optimization, ensuring that images load quickly without sacrificing quality, which is important for SEO. Optimized images improve page speed and enhance the user experience, two critical factors for ranking well.
-
Next.js Image Component: Use the
next/image
component to optimize images automatically. This component provides built-in features like lazy loading, responsive image sizes, and automatic conversion to modern formats (like WebP). -
Alt Text: Ensure that all images have descriptive alt text. This improves accessibility and helps search engines understand the content of your images.
Example usage of next/image
:
import Image from 'next/image';
export default function ProductImage() {
return (
<Image
src="/product.jpg"
alt="Product Name"
width={500}
height={500}
layout="responsive"
/>
);
}
Ranktracker’s Page Speed Insights tool can help assess your image optimization and provide recommendations to improve loading times.
4. Canonical Tags and Managing Duplicate Content
Duplicate content can harm your SEO rankings if search engines find multiple versions of the same content on your site. To prevent this, you should implement canonical tags to signal the primary version of a page.
- Canonical Tags: Use canonical tags to indicate to search engines which URL should be indexed when similar or duplicate content exists. In Next.js, you can add canonical tags using
next/head
.
Example of a canonical tag:
import Head from 'next/head';
export default function ProductPage() {
return (
<Head>
<link rel="canonical" href="https://www.example.com/product" />
</Head>
);
}
Ranktracker’s SEO Audit tool can help detect duplicate content and ensure canonical tags are correctly implemented across your Next.js site.
5. Structured Data and Schema Markup
Implementing structured data using schema markup helps search engines understand your content better and increases the chances of appearing in rich snippets or other enhanced search results.
- JSON-LD: Use JSON-LD to add structured data to your Next.js site. You can inject structured data into your pages using
next/head
or dynamically using API routes.
Common types of structured data for Next.js sites include:
-
Articles: For blog posts and news content.
-
Products: For eCommerce sites displaying products.
-
Breadcrumbs: To show the page’s location within your site’s structure.
Example of JSON-LD for a product page:
import Head from 'next/head';
export default function ProductPage() {
const productSchema = {
"@context": "https://schema.org",
"@type": "Product",
"name": "Product Name",
"description": "A great product description.",
"image": "https://www.example.com/product.jpg",
"sku": "12345",
"brand": {
"@type": "Brand",
"name": "Brand Name"
}
};
return (
<Head>
<script type="application/ld+json">
{JSON.stringify(productSchema)}
</script>
</Head>
);
}
Ranktracker’s SERP Checker can help track how your pages perform in search results and see if they are appearing as rich snippets.
6. XML Sitemaps and Robots.txt
XML sitemaps and robots.txt files are essential for guiding search engines through your site and ensuring they index the right pages.
-
XML Sitemap: Use the
next-sitemap
plugin to automatically generate an XML sitemap for your Next.js site. The sitemap helps search engines discover and crawl your site’s content more efficiently. -
Robots.txt: Create a
robots.txt
file to control which parts of your site search engines should crawl. This file can help prevent search engines from indexing unnecessary or duplicate content.
Install next-sitemap
to generate an XML sitemap:
npm install next-sitemap
Configure the plugin in next-sitemap.config.js
:
module.exports = {
siteUrl: 'https://www.example.com',
generateRobotsTxt: true,
};
Submit your XML sitemap to Google Search Console and monitor how search engines are crawling your Next.js site.
7. Page Speed and Performance Optimization
Next.js is known for its performance optimizations, but there are several steps you can take to ensure your site is as fast as possible. Faster sites rank better, especially on mobile devices.
-
Code Splitting: Next.js automatically splits your JavaScript bundles so that only the necessary code is loaded for each page. This reduces load times and improves performance.
-
Lazy Loading: Use lazy loading for images and components to improve initial page load times.
-
Prefetching: Next.js prefetches pages linked in the background, making navigation between pages faster and seamless for the user.
-
Minify Code: Use the built-in
next.config.js
to minify JavaScript, CSS, and HTML files, reducing file sizes and improving page speed.
Example of enabling code minification in next.config.js
:
module.exports = {
compress: true,
};
Ranktracker’s Page Speed Insights tool can help monitor your site’s load times and suggest improvements to optimize performance.
8. Mobile Optimization and Mobile-First Indexing
With Google’s mobile-first indexing, it’s critical to ensure that your Next.js site is optimized for mobile devices. A fast, responsive site improves user experience and boosts SEO rankings.
- Responsive Design: Ensure that your Next.js site uses responsive
design principles so that it adapts to different screen sizes.
- Mobile Page Speed: Optimize for fast load times on mobile by reducing file sizes, using efficient image formats, and minimizing the use of large, render-blocking scripts.
Ranktracker’s Mobile SEO tool provides insights into how your Next.js site performs on mobile devices and highlights areas for improvement.
9. Analytics and Performance Tracking
To track the success of your SEO efforts, it’s important to integrate analytics tools with your Next.js site.
- Google Analytics: Use the
next/script
component to add Google Analytics tracking to your Next.js site. This allows you to track key metrics such as page views, user behavior, and conversion rates.
Example of adding Google Analytics tracking:
import Script from 'next/script';
export default function MyApp({ Component, pageProps }) {
return (
<>
<Script
src="https://www.googletagmanager.com/gtag/js?id=GA_TRACKING_ID"
strategy="afterInteractive"
/>
<Script id="google-analytics" strategy="afterInteractive">
{`
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'GA_TRACKING_ID');
`}
</Script>
<Component {...pageProps} />
</>
);
}
How Ranktracker Can Help with Next.js SEO
While Next.js provides excellent performance and SEO features out of the box, Ranktracker offers a suite of tools to help you monitor, optimize, and improve your SEO strategy:
-
Keyword Finder: Discover the most relevant keywords for your Next.js pages to target high-traffic search terms.
-
Rank Tracker: Monitor how well your Next.js site is performing in search engine rankings over time and track keyword performance.
-
SEO Audit: Identify technical SEO issues such as slow-loading pages, broken links, or missing metadata that could be hurting your rankings.
-
Backlink Monitor: Track your site’s backlinks to ensure you’re building a strong, authoritative link profile that supports your SEO efforts.
-
SERP Checker: Analyze how your Next.js pages are performing in search results and compare your rankings to your competitors.
Conclusion
Optimizing Next.js SEO involves leveraging the framework’s SSR, SSG, and performance capabilities while following best practices for on-page SEO, structured data, page speed, and mobile optimization. By focusing on these key areas, you can ensure that your Next.js site ranks well in search results and delivers an exceptional user experience.
Pairing Next.js with Ranktracker’s SEO tools provides a comprehensive solution to monitor and improve your site’s performance, helping you achieve long-term success in search engine rankings. Whether you’re building a content-heavy site, eCommerce platform, or web application, Ranktracker can help you optimize and track your SEO efforts effectively.